Understanding Lazy Loading and Eager Loading in JPA
When working with JPA (Java Persistence API) and managing entity relationships, it’s crucial to consider how entities are loaded from the database. One common scenario is when you have two entities, such as University and Student, with a one-to-many relationship where a University can have many Students. In this guide, we’ll explore lazy loading and eager loading strategies for loading associated entities in JPA.
Lazy Loading
Lazy loading is a strategy where associated entities are loaded from the database only when they are explicitly accessed. This can be beneficial for performance, especially when dealing with large datasets or when the associated entities are not always needed.
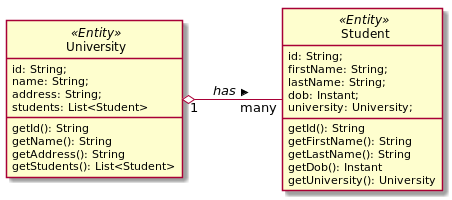
Here’s an example of how to configure lazy loading for the students collection in the University entity:
@Entity
public class University {
@Id
private String id;
private String name;
private String address;
@OneToMany(fetch = FetchType.LAZY)
private List<Student> students;
// getters and setters
}
JavaIn this configuration, the students collection will not be loaded when a University entity is retrieved from the database. Instead, it will be loaded lazily when the getStudents()
method is called.
Eager Loading
In contrast to lazy loading, eager loading is a strategy where associated entities are loaded immediately along with the parent entity. While this can simplify data access in some cases, it may lead to performance issues if the associated entities are large or not always needed.
Here’s an example of how to configure eager loading for the students collection in the University entity:
@Entity
public class University {
@Id
private String id;
private String name;
private String address;
@OneToMany(fetch = FetchType.EAGER)
private List<Student> students;
// getters and setters
}
JavaIn this configuration, the students collection will be loaded eagerly whenever a University entity is retrieved from the database. This means that all associated students will be fetched along with the university data.
LAZY = fetch when needed
EAGER = fetch immediately
Choosing the Right Loading Strategy
The choice between lazy loading and eager loading depends on your specific use case and performance considerations. Here are some guidelines to help you decide:
- Use lazy loading when the associated entities are not always needed or when dealing with large datasets.
- Use eager loading when you consistently need the associated entities along with the parent entity and performance considerations allow for it.
By understanding these loading strategies and configuring your entities accordingly, you can optimize performance and manage data access efficiently in your JPA applications.